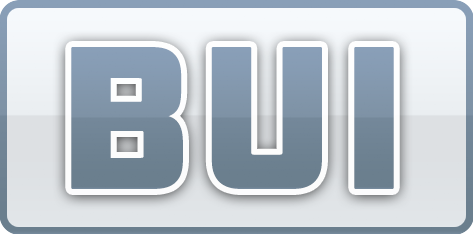
BBjWebManager::setCallback
Description
In BBj 16.00 and higher, this method registers the callback routine for a specified BBjWebManager event.
In BBj 24.11 and higher, this method registers the callback routine for a specified window event. These event type codes are defined by the window interface.
Note:
In BBj 22.03 and higher, BBjWebManager is an alias for BBjBuiManager.
Syntax
Return Value | Method |
---|---|
void | setCallback(int eventType, CustomObject customObject, String methodName) |
void | setCallback(int eventType, String callback) |
int | setCallback(String eventType, String callback) |
int | setCallback(String eventType, String callback, BBjWebEventOptions options) |
int | setCallback(String eventType, CustomObject customObject, String methodName) |
int | setCallback(String eventType, CustomObject customObject, String methodName, BBjWebEventOptions options) |
Parameters
Parameter | Description |
---|---|
eventType | Event type for which the callback is to be registered. |
customObject | A CustomObject containing the method to be called when the event is processed by PROCESS_EVENTS. |
methodName |
The name of the method that is to be called when the event is processed by PROCESS_EVENTS. The method must take a single BBjWebEvent parameter:
|
callback | Subroutine name to be executed in PROCESS_EVENTS. |
options | An optional BBjWebEventOptions structure that specifies JavaScript code to execute inside the client-side event handler and a list of values to return from the event or window. |
Return Value
This method returns the callback ID if the eventType argument is a String.
Remarks
Web Event callbacks can be registered for any string event code. Supported events are defined by the window interface. Internally, this maps to addEventListener.
The setCallback method will implicitly GOSUB to the subroutine specified in subroutineName. Since the block of code executed at callback is arrived at via an implicit GOSUB, a RETURN is required at the end of the subroutine.
The setCallback method will implicitly CALL a program at the specified label when the callback parameter includes "::" (e.g.: "EventHandlerProgram::label"). Since a CALL has been made to a public program, an ENTER may optionally be used at the beginning, and an EXIT is required at the end.
The setCallback method must be called before the PROCESS_EVENTS verb, or within a subroutine that is called in response to an event.
If the setCallback method specifies a non-null BBjWebEventOptions, multiple web event callbacks can be registered for a given eventType. If the setCallback method does not specify a BBjWebEventOptions options parameter, or if the parameter is null, only the last one registered is used, as in BBjControl::setCallback.
Example 1
|
Example 2
|
Version History
- BBj 24.11: Added ways to register the callback routine for a specified window event.
See Also
See the BBj Object Diagram for an illustration of the relationship between BBj Objects.