BBjWindow::addFileChooser
Description
In BBj 7.00 and higher, this method creates a BBjFileChooser in the BBjWindow.
Syntax
Return Value |
Method |
---|---|
addFileChooser(int ID, number x, number y, number w, number h, string directory) |
|
addFileChooser(int ID, number x, number y, number w, number h, string directory, string flags) |
|
BBjFileChooser | addFileChooser(int ID, string directory) |
BBjFileChooser | addFileChooser(int ID, string directory, string flags) |
BBjFileChooser | addFileChooser(string directory) |
BBjFileChooser | addFileChooser(string directory, string flags) |
Parameters
Variable |
Description |
||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
ID |
Specifies the Control ID number. It must be an integer between 1 and 32767 and be unique within a given top level window. |
||||||||||||||||||
x |
Horizontal position of the upper-left corner of the control in current units. |
||||||||||||||||||
y |
Vertical position of the upper-left corner of the control in current units. |
||||||||||||||||||
width |
Width of the control in current units. |
||||||||||||||||||
height |
Height of the control in current units. |
||||||||||||||||||
directory |
Initial directory of the FileChooser |
||||||||||||||||||
flags |
Control flags, as follows:
|
Return Value
Returns the created BBjFileChooser object.
Remarks
A FileChooser control provides an object-oriented, SYSGUI-managed version of the FILEOPEN/FILESAVE functions. Emulate FILESAVE() using the $0100$ flag.
Use the BBjClientFilesystem object in concert with flag $0004$.
If the ID parameter is not specified, a control ID is assigned dynamically using getAvailableControlID().
If the x, y, width, and height parameters are not specified, they are all initialized to 0. This is typically for use with DWC windows that dynamically arrange their contents (window creation flag $00100000$).
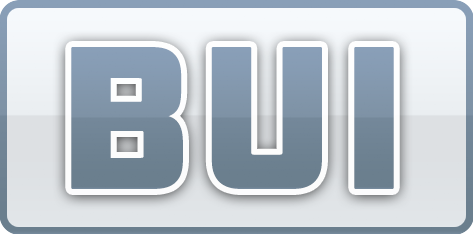
See BUI: Interacting with client files for a detailed discussion of the BUI client-side file choosers.
Example
|
See Also
See the BBj Object Diagram for an illustration of the relationship between BBj Objects.