BBjSysGui::executeScript
Description
In BBj 21.10 and higher, this method executes the specified JavaScript in the client (see Remarks).
Syntax
Return Value | Method |
---|---|
Object | executeScript(String script) |
Object | executeScript(String script, boolean await) |
Parameters
Parameter | Description |
---|---|
script | JavaScript to be executed in the client. |
await | In BBj 23.04 and higher, this optional boolean value specifies that the DWC client should execute this script using the JavaScript await operator. If not specified, the default is false (0). |
Return Value
This method returns the execution result, converted from JavaScript to Java. If the return value is a JavaScript type that can't be represented as a Java type, a simple String representation is returned.
Remarks
In BBj 22.02 and higher, a subset of this functionality is available in the GUI client. Because the client isn't a web page, user-facing components, including Window, Document, and functions like alert, aren't available.
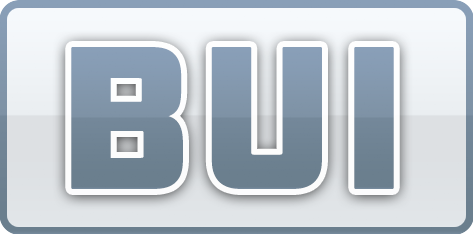
Example
Example 2 (All Clients)
Version History
- BBj 23.04: Added an optional await argument.
See Also
See the BBj Object Diagram for an illustration of the relationship between BBj Objects.